How to link Javascript file to HTML
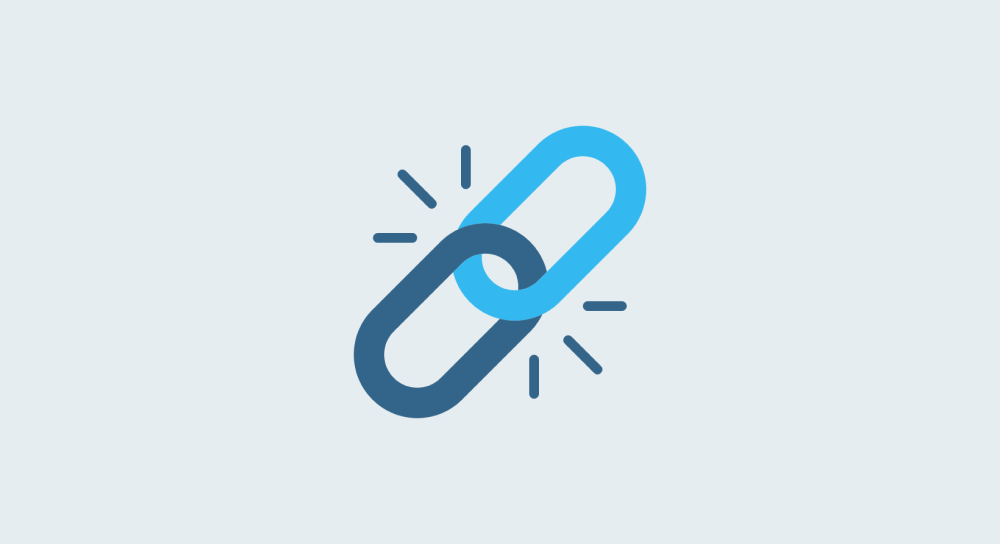
You can link a Javascript file to the HTML document in two easy steps:
Step 1: Create a file that ends with a .js extension
Currently, the browser-supported file extension for the Javascript files is .js
.
So, we have to create a file that ends with .js
extension.
For example: main.js
You can name your Javascript file anything you want
Usually, most people name their Javascript script files as main.js
or custom.js
but you can name your file anything you want.
The above naming convention is a common practice if you are developing a website with only a few Javascript files.
But if you come across the source code of a web application, it is common practice to use app.js
or index.js
because they act as an entry point to other small modules of Javascript files.
Step 2: Link the Javascript file to the HTML document
We use the <script>
tag for this job.
The <script>
tag has an src
attribute, and it accepts a file path to the Javascript file you want to link.
Let's say that you have the following file and folder structure for your project:
.
└── project-folder/
├── index.html
└── js/
└── main.js
If you notice, there is an index.html
file, and the main.js
file is placed inside the js folder.
Here is how you link the main.js
file to the index.html
file.
<!DOCTYPE html>
<html lang="en">
<head>
...
</head>
<body>
<p>Some HTML Content</p>
<script src="js/main.js"></script>
</body>
</html>
In this case, we are providing a relative path of the main.js
file to the HTML document:

What follows is a detailed explanation of relative file paths and absolute file paths and when to use what.
The same rules apply to the .js files with the script tag as well.
So, if you are already good with them, skip to the next lesson directly.
Introducing relative file paths
Let's say that this is the file structure of your HTML project:
.
└── project-folder/
├── index.html
└── main.js
If you notice, both index.html
file and main.js
file are located at the same level inside the file system.
In other words, they sit next to each other on the file system.
Now, let's say we have to link the main.js
to the index.html
using the relative path.
Getting a relative path means asking the following question:
Question: Where is the main.js
file located in relation to the index.html
file?
Answer: It is located at the same level.
Great! If the files are located at the same level, then we just have to provide the filename for the relative path:
<script src="main.js"></script>
With the above relative path, you tell the browser, "Hey! you can find the main.js
file in the same folder as the index.html
file".
Anyway, Let's quickly talk about one more common scenario to have a good understanding of relative paths:
.
└── project-folder/
├── index.html
└── js/
└── main.js
This time, the main.js
file is located inside the js
folder.
So, first, we have to access the js
folder and then access the main.js
file inside it.
And where is the js
folder located in relation to the index.html
file? At the same level, right?
So, here is the relative file path for the main.js
file:
<script src="js/main.js"></script>
Simple, right?
Introducing Absolute file paths
In web browsers, an absolute file path is a full path (full URL) for the file involving the HTTP protocol.
So, an absolute file path starts with the HTTP protocol.
For example, let's just say that the main.js
file is hosted on the assets directory of the usablewp.com
website.
In this case, the absolute file path for the main.js
file would look like this:
<script src="http://www.usablewp.com/assets/main.js"></script>
Now, this is the same as the following:
<script src="/assets/main.js"></script>
If you notice, the above file path is starting with a forward-slash /
instead of the HTTP protocol.
In the world of web browsers, the forward slash /
is the same as the full domain name, including the HTTP protocol:

So, most people will just use the /
pattern instead of entering the full domain name. It is much easier and least error-prone in some server setups.
Relative file path "versus" Absolute file path
Relative paths are recommended when we are developing a website locally.
Absolute paths are recommended when we are using a web server to serve our website files or if we are linking to an external Javascript resource like jQuery.
This explanation will become more clear when we are building our AJAX project because AJAX projects require a web server to function.
After linking the file, write the Javascript code inside the javascript file.
And the browser will take care of the rest.


In the next lesson, we will talk about why you should place the <script>
tags at the bottom of the HTML document.