Data types: String, Number, and Boolean
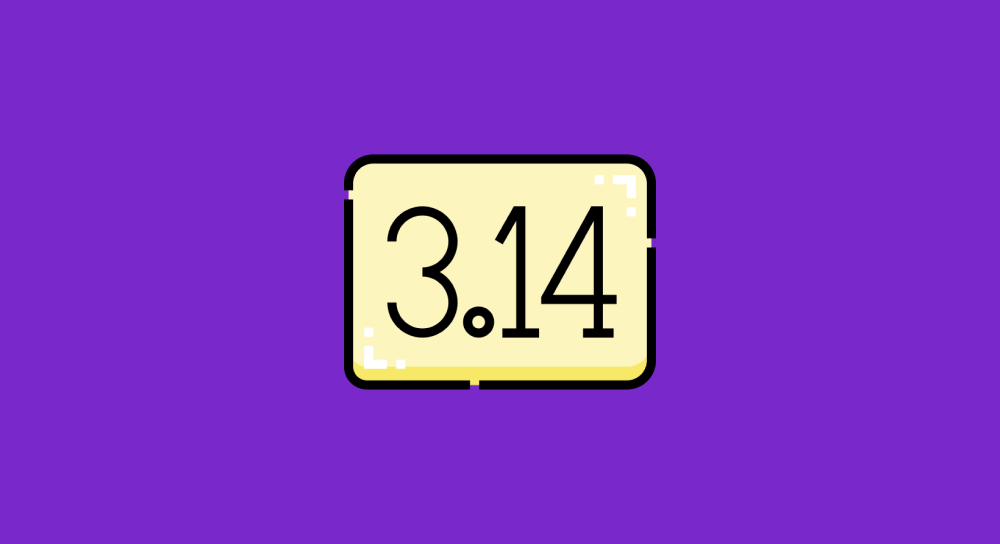
In the last couple of lessons, we learned how to create variables and name them.
Now, let's see what data types you can store inside a variable.
So, what is a data type?
Data simply means a unit of information such as:
- 34 (Age)
- "Naresh Devineni" (Name)
- 4.2 (GPA)
And data in Javascript is always of a certain type:
- 34 - Number
- "Naresh Devineni" - String
- 4.2 - Integer
Currently, there are eight basic data types in JavaScript.
- String
- Number
- Boolean
- null
- undefined
- Objects
- Symbols
- BigInt
In this lesson, we will cover the three most widely used data types quickly:
String
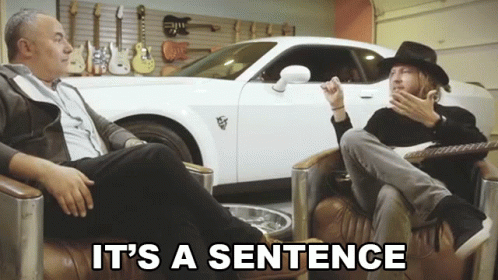
A string is like a sentence.
Language doesn't matter.
In other words, a String represents text data.
For example, a welcome message:
let welcomeMessage = "Heylo! Welcome to Mini Lessons.";
or a name:
let name = "Naresh Devineni";
If you notice the above example, the string value is surrounded by quotes.
The thing is, a string
must be surrounded by quotes in Javascript.
The quotes can be a single quote or a double quote. It doesn't matter. They both mean the same to Javascript.
In fact, there are three types of quotes in Javascript:
- Single Quotes -
'Hello'
- Double Quotes -
"Hello"
- Backticks -
`Hello`
// Double Quotes
let welcomeMessage = "Heylo! Welcome to Mini Lessons.";
// Single Quotes
let thankYouMessage = 'Thanks for contacting us!';
// Backticks
let name = "MiniLessons";
let dynamicWelcomeMessage = `Welcome ${name}! How can we help you?`;
Backticks have a special capability compared to single and double quotes because backticks allow you to build dynamic strings.
We will learn more about backticks and dynamic strings in a future lesson.
A String can also be empty. For example, when declaring a variable, we can give an empty string as a value.
let salaryIncrement = ""; // Not sure yet :P
An empty string means "Quotes with no characters in between".
Number
A number can be an integer or a floating-point number.
For example:
// Postive integer
let age = 34;
// Negative integer
let marks = -45;
// Fraction
let pi = 3.141592653589793238;
You can perform mathematical operations on numbers in Javascript.
For example, addition +
, subtraction -
, multiplication *
, division /
, etc.
If you surround a number with quotes, Javascript will treat it as a string.
let age = "34"; // The number 34 is now technically a string
And you can not perform mathematical operations on strings.
Special Numeric Values
Apart from integers and floating-point numbers, three more numeric values are considered numbers in Javascript.
Infinity
- represents the highest possible positive number-Infinity
- represents the highest possible negative numberNaN
- Not a Number
NaN
(Not a Number) as a number.Usually, NaN
is the result when you try to perform a mathematical operation that is not valid.
For example, trying to divide a string with a number will result in NaN
.

Any line of code that results in NaN
will cause a lot of bugs in your code.
When coding mathematical-related projects, you'll often see NaN
the result of invalid input somewhere in the code.
So, you must be careful when performing some math operations.
We will be discussing more about the NaN
value in future lessons.
But we will not discuss the usage of Infinity
and -Infinity
in this course.
Boolean
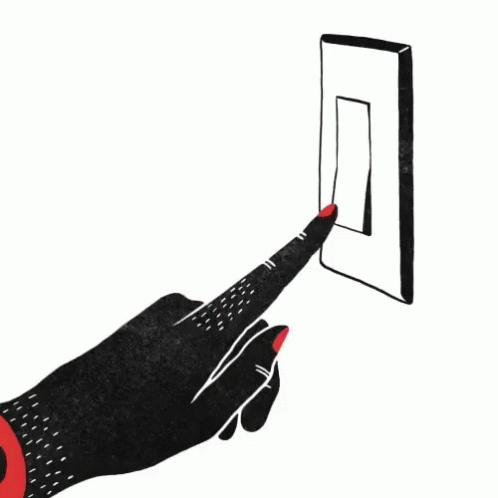
Boolean can be easily understood by comparing it with the switch of a light bulb.
At any point in time, a bulb can be switched on or off. But not both at the same time.
Another example is a court verdict. Once all the evidence is examined, a court judge will declare whether the accused is either guilty or not guilty.
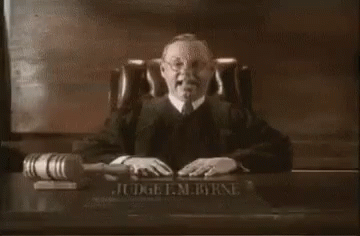
Similarly, a Boolean can only have two values, true or false.
That means you can assign the keywords true
or false
as a value for a variable.
For example:
let are_you_eligible_for_voting = true;
let doYouSmoke = false;
let areYouMarried = true;
Again, unlike strings, boolean values must not be surrounded by quotes.
The Conclusion
String
, Number
and Boolean
are the most used data types in Javascript.
Trust me, you can write a lot of good Javascript programs using just these three data types only.
So, it is important you understand these data types in a good way.
And to ensure that, in the next lesson, we will perform a small exercise to help you remember what you have learned about variables and data types so far.
Ready player one?