Coding styles and readability
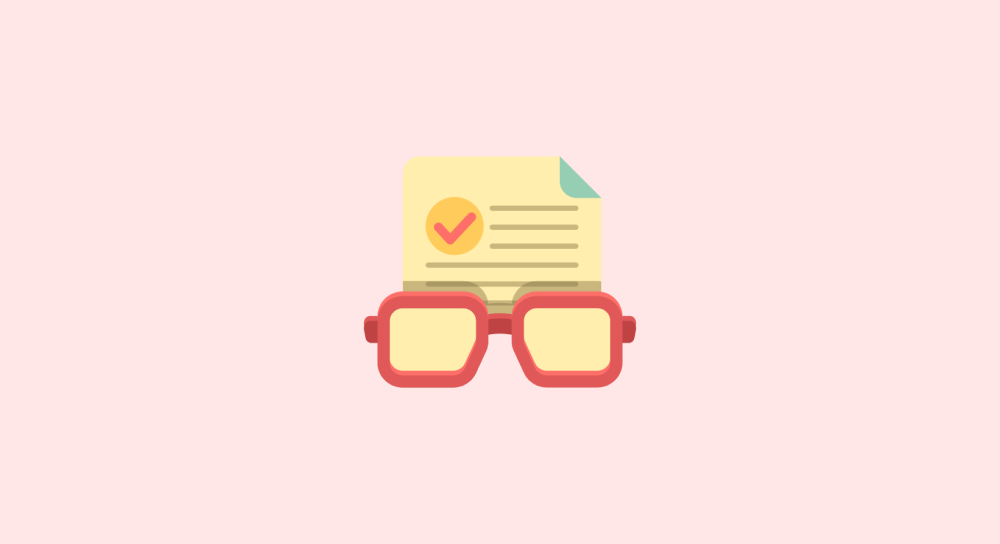
Coding Styles
There are multiple coding styles for declaring and using variables.
We can also declare and assign values to multiple variables in the same line:
let name = "Naresh Devineni", age = 34, education = "Computer Science";
If you notice, the let
keyword is used only once for creating all three variables.
Every programmer is a different person.
So, the coding styles slightly differ as well.
For example, sometimes, you might come across variables declared like this:
//Declaring variables in a single line
let name, age, education;
//But assigning values to them in seperate lines
name = "Naresh Devineni";
age = 34;
education = "Computer Science";
Or in a multi-line style:
//Declaring variables in a single line
let name = "Naresh Devineni",
age = 34,
education = "Information Technology";
Or even a multi-line comma-first style:
//Declaring variables in a single line
let name = "Naresh Devineni"
, age = 34,
, education = "Information Technology";
All these variants do the same thing, and they are all valid Javascript.
So, it all boils down to personal preference.
Having said that...
Code readability is important.
Tell me something.
Here is code snippet 1, where the code is rammed into a single line:
//Code rammed into a single line
let name = "Naresh Devineni", age = 34, education = "Computer Science";
And here is code snippet 2:
//Code is separated into multiple lines
let name = "Naresh Devineni";
let age = 34;
let education = "Computer Science";
They both do the same job of creating variables.
But which snippet do you think is easier to read?

Snippet 1 is concise but snippet 2 looks neat and readable!
Nice, Good Answer!
When you are writing code, you shouldn't just think about yourself.
You might like snippet 1 because it is clear and concise for you:
let name = "Naresh Devineni", age = 34, education = "Computer Science";
But sometimes, the code we write could be used by our colleagues or people on the internet.
They need to read and understand your code to use it effectively.
And the only way you can make their life easier is by writing readable code like snippet 2:
let name = "Naresh Devineni";
let age = 34;
let education = "Computer Science";
Now, this does not just apply to declaring variables.
In your journey as a programmer, you'll come across a lot of coding styles that save you a few lines of code but end up hurting the readability.
So always make conscious decisions about the readability when you are using a particular coding style.
In the next lesson, we will learn about various types of data you can store inside variables.